Home→Forums→MonoBrick EV3 Firmware→I2C communication PCF8574AP→Reply To: I2C communication PCF8574AP
October 9, 2014 at 14:33
#4873
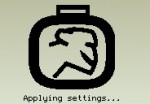
Bartłomiej Drozd
Participant
sorry, i don’t know how to read a register… in NXC i did it that:
void writeReg(byte reg)
{
byte buff[] = {0x70, 0x00};
buff[1] = reg;
int nbytes;
I2CWrite(I2C, 0, buff);
while(I2CStatus(I2C, nbytes) == STAT_COMM_PENDING);
Wait(100);
}
because i need only outputs and that is working fine, but i need it in C# on EV3. Maybe i’m doing something wrong? Here is my code…
Main.cs
using System;
using System.Threading;
using MonoBrickFirmware;
using MonoBrickFirmware.Display.Dialogs;
using MonoBrickFirmware.Display;
using MonoBrickFirmware.Sensors;
using MonoBrickFirmware.UserInput;
namespace test
{
class MainClass
{
public static void Main (string[] args)
{
EventWaitHandle stopped = new ManualResetEvent (false);
ButtonEvents btn = new ButtonEvents ();
byte address = 0x70;
byte[] data1 = new byte[8] {1, 1, 1, 1, 1, 1, 1, 1};
byte[] data0 = new byte[8] {0, 0, 0, 0, 0, 0, 0, 0};
LcdConsole.WriteLine ("hello world!");
pcf pcf = new pcf (SensorPort.In1, address);
btn.EscapePressed += () => {
LcdConsole.WriteLine("stopped");
stopped.Set ();
};
btn.LeftPressed += () => {
pcf.WriteReg(0, data1);
LcdConsole.WriteLine("write data1");
};
btn.RightPressed += () => {
pcf.WriteReg(0, data0);
LcdConsole.WriteLine("write data0");
};
stopped.WaitOne ();
}
}
}
pcf.cs
using System;
using MonoBrickFirmware;
using MonoBrickFirmware.Sensors;
namespace test
{
public class pcf : I2CSensor
{
public pcf (SensorPort Port, byte adress) : base (Port, adress, I2CMode.LowSpeed)
{
base.Initialise();
}
public byte[] ReadReg(byte Register, byte Length)
{
return base.ReadRegister(Register, Length);
}
public void WriteReg(byte Register, byte[] Data)
{
base.WriteRegister(Register, Data);
}
public byte[] ReadAndWriteReg(byte Register, byte[] Data, int rxLength)
{
return base.WriteAndRead(Register, Data, rxLength);
}
public override string ReadAsString()
{
throw new NotImplementedException();
}
public override void SelectNextMode()
{
throw new NotImplementedException ();
}
public override string GetSensorName()
{
return "PCF8574AP";
}
public override void SelectPreviousMode()
{
throw new NotImplementedException ();
}
public override int NumberOfModes()
{
return 0;
}
public override string SelectedMode()
{
return "0";
}
}
}
Follow