Home→Forums→MonoBrick Communication Library→Trigger brick program from Python→Reply To: Trigger brick program from Python
January 6, 2014 at 22:19
#3781
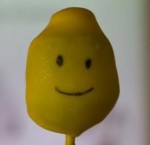
Anders Søborg
Keymaster
Although this forum is related to MonoBrick communication here are some Phyton code to send a message to the EV3 from a PC over Bluetooth
import struct
import ctypes
import binascii
import socket
import time
import StringIO
import serial
import struct
from array import array
from serial import *
from threading import Thread
class MailboxMessage():
def __init__(self, mailboxName, msg):
self.MailboxName = mailboxName
self.msg = msg
mailboxNameLength = len(mailboxName)+1
msgLength = len(msg) +1
totalLength = mailboxNameLength + msgLength + 7
self.data = bytearray(b"")
self.data.append(totalLength)#length
self.data.append(0)
self.data.append(100)#sequence number
self.data.append(0)
self.data.append(129)#Command type - system command without reply 0x81
self.data.append(158)#system command type - WriteMailbox 0x9e
self.data.append(mailboxNameLength)
self.data = self.data + bytearray(mailboxName)
self.data.append(0)
self.data.append(msgLength)
self.data.append(0)
self.data = self.data+ bytearray(msg)
self.data.append(0)
def Mailbox(self):
return self.MailboxName
def Data(self):
return self.data
def Message(self):
return self.msg
class Receiver(Thread):
def __init__(self, serialPort):
Thread.__init__(self)
self.serialPort = serialPort
def run(self):
message = []
messageSize=0
while True:
for c in self.serialPort.read(size=1):
message.append(ord(c))
if len(message) == 2:
bytes = array('B', message)
messageSize = struct.unpack('<H', bytes )[0]
#print messageSize
for c in self.serialPort.read(size=messageSize):
message.append(ord(c))
bytes = array('B', message[6:7])
mailboxNameLength = struct.unpack('<B', bytes )[0]
#bytes = array('B', message[7+mailboxNameLength:7+mailboxNameLength+2])
#mailboxTextLength = struct.unpack('<H', bytes )[0]
mailboxName = array('B', message[7:7+mailboxNameLength-1]).tostring()
mailboxText = array('B', message[7+mailboxNameLength+2 :messageSize+1]).tostring()
mailboxMessage = MailboxMessage(mailboxName, mailboxText)
print mailboxMessage.Mailbox()
print mailboxMessage.Message()
message[:] = []
self.serialPort.close()
class Sender(Thread):
def __init__(self, serialPort):
Thread.__init__(self)
self.serialPort = serialPort
def run(self):
text = ""
print "Enter text to send"
while(text != "quit\n"):
msg = raw_input("")
mailboxName = "mailbox1"
self.sendMessage(mailboxName, msg)
self.serialPort.close()
def sendMessage(self, mailboxName,msg):
mailboxMessage = MailboxMessage(mailboxName,msg)
self.serialPort.write(mailboxMessage.Data())
serialPort = Serial(port='/dev/tty.EV3-SerialPort', baudrate=9600)
send = Sender(serialPort)
receive = Receiver(serialPort)
send.start()
receive.start()
To start a program you need to change the command to be a start program command. You can find more info on this and other direct commands here.
Anders
Follow