Home→Forums→MonoBrick EV3 Firmware→How to use AbsoluteIMU-ACG sensor?→Reply To: How to use AbsoluteIMU-ACG sensor?
October 31, 2014 at 17:43
#4945
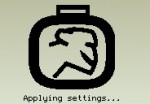
Tcm0
Participant
Could you please try the following code?
using System;
using MonoBrickFirmware.Sensors;
namespace MonoBrickHelloWorld
{
public class MindsensorsAbsoluteIMU : I2CSensor
{
public MindsensorsAbsoluteIMU (SensorPort Port) : base (Port, (byte)0x22, I2CMode.LowSpeed)
{
base.Initialise ();
}
public void ChangeAccelerometerSensitivity (string Sensitivity)
{
byte[] BytesToWrite = {(byte)0};
if (Sensitivity == "2G" || Sensitivity == "2g") BytesToWrite[0] = (byte) 0x31;
else if (Sensitivity == "4G" || Sensitivity == "4g") BytesToWrite[0] = (byte) 0x32;
else if (Sensitivity == "8G" || Sensitivity == "8g") BytesToWrite[0] = (byte) 0x33;
else if (Sensitivity == "16G" || Sensitivity == "16g") BytesToWrite[0] = (byte) 0x34;
else throw new ArgumentException();
base.WriteRegister((byte)0x22, BytesToWrite);
return;
}
public byte[] ReadX ()
{
return(base.ReadRegister ((byte)0x42, 8));
}
public byte[] ReadY ()
{
return(base.ReadRegister ((byte)0x43, 8));
}
public byte[] ReadZ ()
{
return(base.ReadRegister ((byte)0x44, 8));
}
public override string ReadAsString()
{
return("X: " + Convert.ToString(base.ReadRegister(0x42)) + " Y: " + Convert.ToString(base.ReadRegister(0x43)) + " Z: " + Convert.ToString(base.ReadRegister(0x44)));
}
public override void SelectNextMode()
{
return;
}
public override void SelectPreviousMode()
{
return;
}
public override string GetSensorName()
{
return (Convert.ToString (base.ReadRegister ((byte)0x10, (byte)0x07)));
}
public override int NumberOfModes()
{
return 1;
}
public override string SelectedMode()
{
return ("Mode 1");
}
}
}
using System;
using MonoBrickFirmware;
using MonoBrickFirmware.Display;
using MonoBrickFirmware.UserInput;
using System.Threading;
using MonoBrickFirmware.Sensors;
namespace MonoBrickHelloWorld
{
class MainClass
{
public static void Main (string[] args)
{
EventWaitHandle stopped = new ManualResetEvent (false);
MindsensorsAbsoluteIMU NewSensor = new MindsensorsAbsoluteIMU (SensorPort.In1);
ButtonEvents buts = new ButtonEvents ();
buts.EscapePressed += () => {
stopped.Set ();
};
buts.EnterPressed += () => {
LcdConsole.WriteLine(NewSensor.ReadAsString());
};
LcdConsole.WriteLine ("Hello World");
stopped.WaitOne ();
}
}
}
(Why can’t we attach .cs files?)
-
This reply was modified 9 years, 9 months ago by
Tcm0.
Follow