Home→Forums→MonoBrick EV3 Firmware→interface an Arduino Uno as an i2c slave to EV3?→Reply To: interface an Arduino Uno as an i2c slave to EV3?
June 22, 2014 at 10:28
#4449
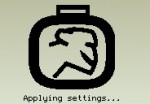
Tcm0
Participant
Nevermind, I got it: Klick me.
Here is my wiring diagram (yes, my breadboard is a mess): Klick me.
And now the code.
My Arduino class:
using System;
using MonoBrickFirmware;
using MonoBrickFirmware.Sensors;
namespace EV3Temeplate
{
class Arduino : I2CSensor
{
public Arduino(SensorPort Port, byte adress)
: base (Port, adress, I2CMode.LowSpeed)
{
base.Initialise();
}
public byte[] ReadReg(byte Register, byte Length)
{
return base.ReadRegister(Register, Length);
}
public void WriteReg(byte Register, byte[] Data)
{
base.WriteRegister(Register, Data);
}
public byte[] ReadAndWriteReg(byte Register, byte[] Data, int rxLength)
{
return base.WriteAndRead(Register, Data, rxLength);
}
public override string ReadAsString()
{
throw new NotImplementedException();
}
}
}
My program.cs:
using System;
using System.Threading;
using MonoBrickFirmware;
using MonoBrickFirmware.Display;
using MonoBrickFirmware.Sensors;
using MonoBrickFirmware.UserInput;
namespace EV3Temeplate
{
class Program
{
static void Main(string[] args)
{
EventWaitHandle stopped = new ManualResetEvent(false);
LcdConsole.WriteLine("Connecting...");
byte Adresse = 0x04 << 1;
ButtonEvents buts = new ButtonEvents();
Arduino Arduino1 = new Arduino(SensorPort.In1, Adresse);
byte[] Ergebnis = new byte[1];
LcdConsole.WriteLine("Connected");
LcdConsole.WriteLine("Press Enter to read I2C Port");
LcdConsole.WriteLine("Press Escape to leave program");
buts.EscapePressed += () => {
stopped.Set();
};
buts.EnterPressed += () => {
Ergebnis = Arduino1.ReadReg(0x04, 1);
LcdConsole.WriteLine("Result: " + Convert.ToString(Ergebnis[0]));
};
stopped.WaitOne();
}
}
}
and the program that runs on the Arduino (sorry, I can’t organize it): Klick me.
Follow